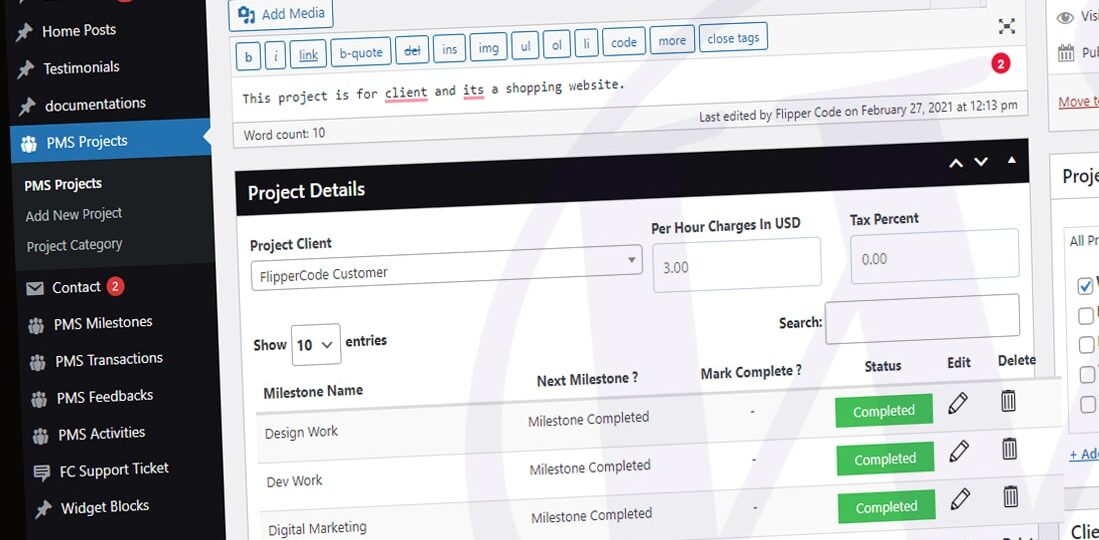
Meta boxes are the small piece of HTML to add on administrative interface. These are used to extend posts/pages or custom post type edit screens to ask more related information.
Using meta boxes, you can organized custom fields in a better way.
How to Add Meta Boxes
We’ve discussed hooks in details. We’ll use add_meta_boxes action here to add a meta box to add or edit posts screens. Below is example output of our tutorial.

Let’s try to understand this in steps.
- Step 1: We need to register a hook “add_meta_boxes” first.
add_action('add_meta_boxes','func_extra_fields');
- Step 2: In the definition of “func_extra_field”, we’ll use “add_meta_box” function to pass required parameters to display a form on the ‘post’ screens.
function func_extra_fields() { add_meta_box("extra_fields","Extra Fields","func_custom_forms","post"); }
- Step 3: “func_custom_forms” is a callback function for add_meta_box which is responsible for the output of the form.
function custom_forms() { wp_nonce_field( 'post_inner_custom_box', 'post_inner_custom_box_nonce' ); $output="
These are extra fields added by add_meta_box function.
; input type='text' value='' id='txt_field' name='txt_field'>"; echo $output; }
Note that we didn’t use any submit button here because default ‘publish’ button will be used to save this data.
- Step 4: Now we need to save this data when click on publish button. For this, we’ll need to handle ‘save_post’ action which is triggered when a new blog post created or saved by clicking on publish button.
add_action('save_post','func_save_data_now');
We need to write our form data handling code in func_save_data_now function.
function func_save_data_now() { if ( ! isset( $_POST['post_inner_custom_box_nonce'] ) ) return $post_id; $nonce = $_POST['post_inner_custom_box_nonce']; // Verify that the nonce is valid. if ( ! wp_verify_nonce( $nonce, 'post_inner_custom_box' ) ) return $post_id; if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE ) return $post_id; if ( 'page' == $_POST['post_type'] ) { if ( ! current_user_can( 'edit_page', $post_id ) ) return $post_id; } else { if ( ! current_user_can( 'edit_post', $post_id ) ) return $post_id; } $txt_field = sanitize_text_field( $_POST['custom_txt_field'] ); update_post_meta( $post_id, 'custom_txt_field', $txt_field ); }
Conclusion
So idea is that every field we add using add_meta_box save as post meta data using update_post_meta. ‘update_post_meta’ will call ‘add_post_meta’ if meta key doesn’t exists. Before using update_post_meta, we added few essential checklist validations which are necessary whenever you save anything in the database.
Explore the latest in WordPress
Trying to stay on top of it all? Get the best tools, resources and inspiration sent to your inbox every Wednesday.